¶ Authing OTP
The current OTP SDK we provide supports TOTP(The Time-based One-time Password algorithm), Can be used to Multifactor authentication(MFA) (opens new window) in the OTP authentication method. The SDK can quickly generate the OTP verification code and provide the ability to add, delete, modify, and check. You can also download Authing OTP (opens new window) Experience the official Authing application.
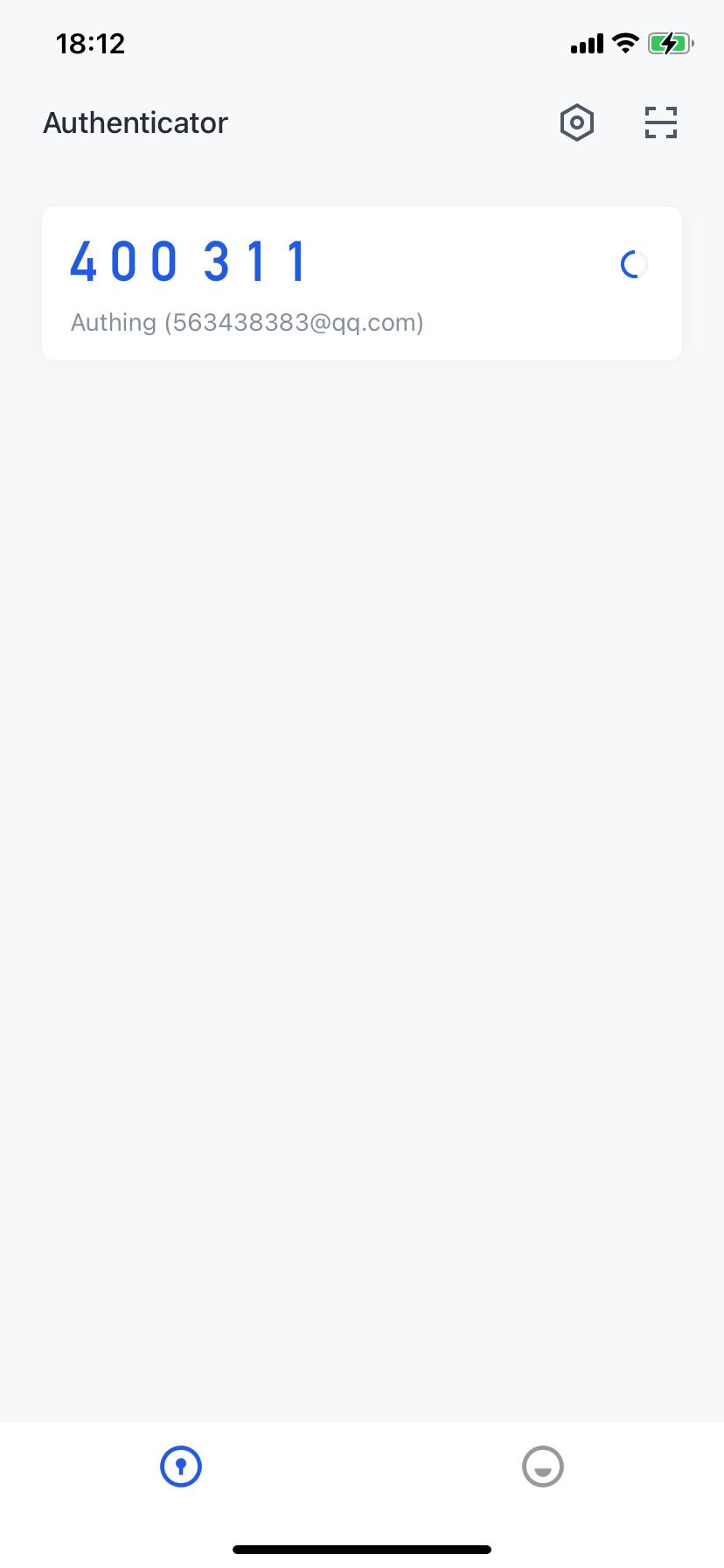
¶ Data format
The OTP data format is uri, for example:
otpauth://totp/GuardPool:test?secret=E55EYQJ5EUHX6ALB&period=30&digits=6&algorithm=SHA1&issuer=GuardPool
After parsing through the URI
class:
scheme
otpauthhost
totppath
GuardPool:testquery
secret=E55EYQJ5EUHX6ALB&period=30&digits=6&algorithm=SHA1&issuer=GuardPool
¶ Add dependency
Add sdk dependencies in dependencies
in the project build.gradle file:
implementation 'cn.authing:otp:1.0.2'
¶ TOTPEntity class
TOTP account entity class, the parameters are as follows:
Name | Type | Description |
---|---|---|
path | String | Path, example:"GuardPool:test" |
application | String | Name of organization or application, example:"GuardPool" |
account | String | Account name, example:"test" |
secret | String | Secret, example:"E55EYQJ5EUHX6ALB" |
period | int | Coken interval, In seconds, example:30s |
digits | int | Secret digit, example:6 |
algorithm | String | algorithm, example:"SHA1"、"SHA256"、"SHA512" |
issuer | String | issuer, example:"GuardPool" |
totpCode | String | code, example:"888888" |
If the secret
、period
、digits
、algorithm
are set, TOTP code
can be obtained directly through the getTotpCode()
method.。
¶ TOTP class
TOTP Account data management class.
¶ Add data
Raw data binding can be passed directly through the bind()
method
public static TOTPBindResult bind(Context context, String data)
example:
String otpData = "otpauth://totp/GuardPool:test?secret=E55EYQJ5EUHX6ALB&period=30&digits=6&algorithm=SHA1&issuer=GuardPool";
TOTPBindResult bindResult = TOTP.bind(this, otpData);
if (bindResult.getCode() == TOTPBindResult.BIND_SUCCESS) {
//Binding success
} else if (bindResult.getCode() == TOTPBindResult.UPDATED_ACCOUNT) {
//Update account
} else if (bindResult.getCode() == TOTPBindResult.BIND_FAILURE) {
//Binding failure
}
It can also be added via the addTotp()
method
example:
OTPEntity totp = new TOTPEntity();
totp.setPath("GuardPool:test");
totp.setApplication("GuardPool");
totp.setAccount("test");
totp.setSecret("E55EYQJ5EUHX6ALB");
totp.setAlgorithm("SHA1");
totp.setDigits(6);
totp.setPeriod(30);
totp.setIssuer("GuardPool");
TOTP.addTotp(this, totp);
¶ Update data
Update data
public static void updateTotp(Context context, TOTPEntity totp)
¶ Obtain data
Get all the data
public static List<TOTPEntity> getTotpList(Context context)
Get a single piece of data through path
public static TOTPEntity getTotp(Context context, String path)
¶ Delete data
Delete via entity class
public static void deleteTotp(Context context, TOTPEntity totp)
Delete by path
public static void deleteTotp(Context context, String path)
¶ TOTPGenerator class
TOTP verification code generation class, TOTP code
can be generated by generateTOTP()
method of the TOTPGenerator class itself
public static String generateTOTP(String secret, int period, int digits, String algorithm)
¶ Safety suggestion
For the sake of security, it is recommended to prohibit screenshots on the interface displaying code
and add the following code:
getWindow().addFlags(WindowManager.LayoutParams.FLAG_SECURE);